Spiral waveguide
SiFab contains three spirals:
FixedPortWithLengthSpiral: a spiral where the position of the ports and the length can be easily controlled by the user.
DoubleSpiralFixedBend: a double spiral with fixed bends
DoubleSpiralWithInCouplingFixedBend: a double spiral with fixed bends and and in coupling region
FixedLengthSpiralFixedBend: a spiral with a fixed length and fixed bends
FixedPortWithLengthSpiral
This class allows to connect two ports with a fixed position (input_port
and output_port
) using a spiral.
The total port-to-port spiral length (total_length
) and the number of loops (n_o_loops
) can be fully controlled by the user.
Alternatively to using two ports with fixed position, the user can specify
a fixed input port (
input_port
) and the distance between the ports (ports_distance
), orthe distance between the ports (
ports_distance
) without specifying any port. In this case,input_port
is placed at the origin.
By default, the spiral is drawn starting 100 um from the input port (along the vector connecting the input and the output ports), however this can be adapted by changing the value of offset_spiral_start
.
If a layout with the given property values cannot be generated, an exception is raised with suggestions on how to meet the layout specifications.
Reference
Click on the name of the component below to see the complete PCell reference.
Spiral with fixed position for the ports or a fixed length. |
Example
from si_fab import all as pdk
from ipkiss3 import all as i3
p1 = i3.OpticalPort(
name="in",
position=(0.0, 0.0),
angle_deg=0.0,
trace_template=pdk.SiWireWaveguideTemplate(),
)
p2 = i3.OpticalPort(
name="out",
position=(400.0, 0.0),
angle_deg=180.0,
trace_template=pdk.SiWireWaveguideTemplate(),
)
sp = pdk.FixedPortWithLengthSpiral(
input_port=p1,
output_port=p2,
total_length=5000.0,
n_o_loops=5,
)
sp_lv = sp.Layout()
sp_lv.visualize(annotate=True)
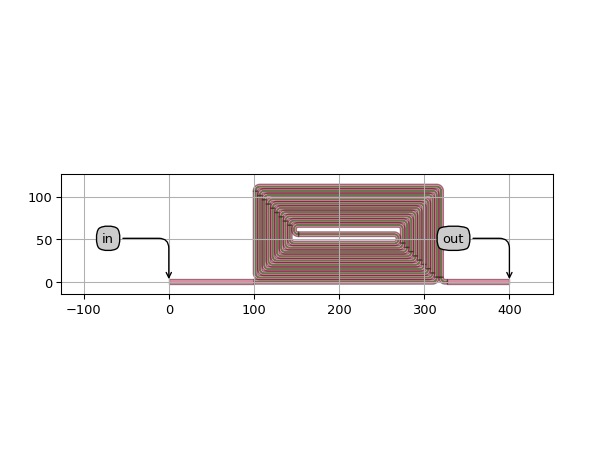
DoubleSpiralFixedBend
This class is used to create a double spiral with a fixed bend cell that can be re-used for each bend of the spiral.
This bend cell that is provided can be defined by the user.
The user can also provide the trace template for the straight sections, the length of the tapers and the minimum length of the straight sections both between and before the tapers.
Otherwise, this PCell works identically to the DoubleSpiral
class from Picazzo.
Reference
Click on the name of the component below to see the complete PCell reference.
|
Example
from si_fab import all as pdk
from ipkiss3 import all as i3
trace_template = pdk.SiWireWaveguideTemplate()
trace_template.Layout(core_width=0.5, cladding_width=6.0)
bend = pdk.WaveguideBend(trace_template=trace_template)
bend.Layout(bend_size=5.0, bend_radius=5.0, rounding_algorithm=i3.ShapeRound)
spiral = pdk.DoubleSpiralFixedBend(bend=bend, n_o_loops=5)
spiral_lo = spiral.Layout()
spiral_lo.visualize()
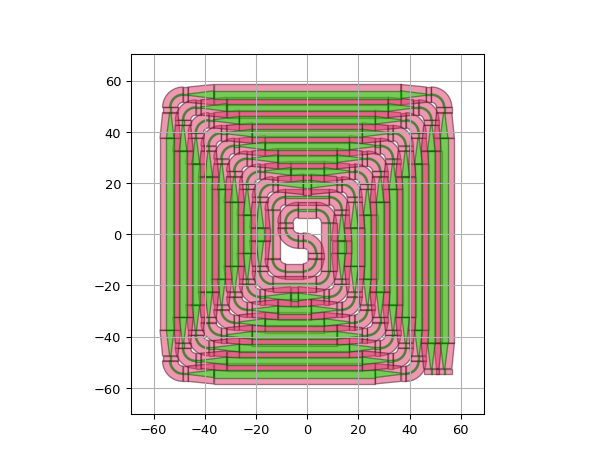
DoubleSpiralWithInCouplingFixedBend
This class is used to create a double spiral with a fixed bend cell that can be re-used for each bend of the spiral.
This bend cell that is provided can be defined by the user.
The user can also provide the trace template for the straight sections, the length of the tapers and the minimum length of the straight sections both between and before the tapers.
Otherwise, this PCell works identically to the DoubleSpiralWithInCoupling
class from Picazzo.
Reference
Click on the name of the component below to see the complete PCell reference.
|
Example
from si_fab import all as pdk
from ipkiss3 import all as i3
trace_template = pdk.SiWireWaveguideTemplate()
trace_template.Layout(core_width=0.5, cladding_width=6.0)
bend = pdk.WaveguideBend(trace_template=trace_template)
bend.Layout(bend_size=5.0, bend_radius=5.0, rounding_algorithm=i3.SplineRoundingAlgorithm())
spiral = pdk.DoubleSpiralWithInCouplingFixedBend(bend=bend, n_o_loops=5)
spiral_lo = spiral.Layout()
spiral_lo.visualize()
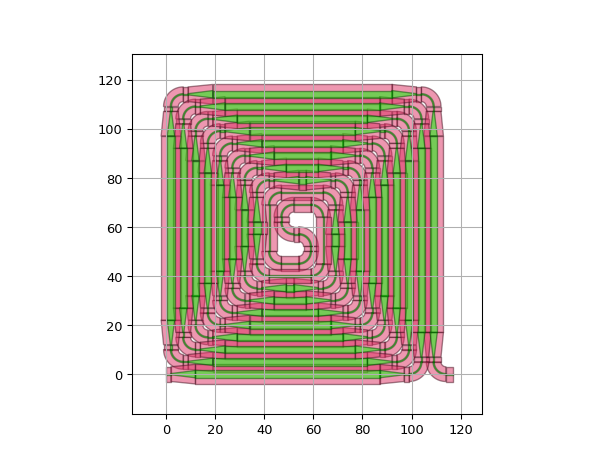
FixedLengthSpiralFixedBend
This class is used to create a double spiral with a fixed length and a fixed bend cell that can be re-used for each bend of the spiral.
This bend cell that is provided can be defined by the user.
The user can also provide the trace template for the straight sections, the length of the tapers and the minimum length of the straight sections both between and before the tapers.
Otherwise, this PCell works identically to the FixedLengthSpiral
class from Picazzo.
Reference
Click on the name of the component below to see the complete PCell reference.
|
Example
from si_fab import all as pdk
bend = pdk.EulerFixedBend()
spiral = pdk.FixedLengthSpiralFixedBend(bend=bend, total_length=4000, n_o_loops=4)
spiral_lo = spiral.Layout(
incoupling_length=10.0,
spacing=7,
stub_direction="V", # either H or V
growth_direction="V",
)
spiral_lo.visualize()
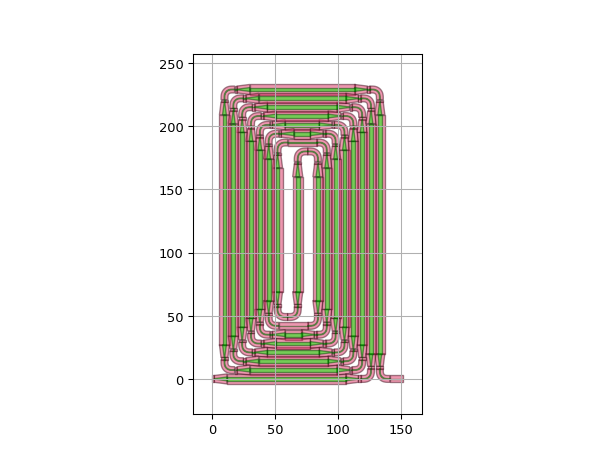