Note
Go to the end to download the full example code
Simulating an aperture with CAMFR
This simple example illustrates the integration of SlabSim in IPKISS. It requires the Luceda AWG Designer module. We make a simple aperture and run a CAMFR simulation on it.
Note: do not use this aperture in a real design, this is only for illustration purposes and not designed optimally.
Getting started
We start by importing the technology with other required modules:
from si_fab import all as pdk
import ipkiss3.all as i3
import numpy
From IPKISS’ AWG Designer and si_fab_awg, we import the following:
from awg_designer.all import SimpleSlabMode
from si_fab_awg.all import SiRibAperture, SiSlabTemplate
Template for the free propagation region. This defines the layers, slab modes, etc.
slab_t = SiSlabTemplate()
slab_t.Layout()
slab_t.SlabModes(modes=[SimpleSlabMode(name="TE0", n_eff=2.8, n_g=3.2, polarization="TE")])
Specify the wavelength of interest in an Environment object
environment = i3.Environment(wavelength=1.55)
The aperture
Make an aperture consisting of a transition between two trace templates. Trace template at the aperture
ap_wg_t = pdk.SiRibWaveguideTemplate()
ap_wg_t.Layout(core_width=2.0)
Trace template at the waveguide port
wg_t = pdk.SiWireWaveguideTemplate()
wg_t.Layout(core_width=0.4)
The aperture
ap = SiRibAperture(
slab_template=slab_t,
aperture_trace_template=ap_wg_t,
trace_template=wg_t,
taper_length=30,
)
ap_lo = ap.Layout() # the length is calculated by default
ap_lo.visualize()
ap_lo.visualize_2d()
Get the field profile at the aperture
This will execute a CAMFR simulation. By putting verbose=True, the simulation prints the wavelength and effective indices used for the simulation.
ap_sm = ap.FieldModelFromCamfr()
f = ap_sm.get_fields(environment=environment, verbose=True)
f.visualize()
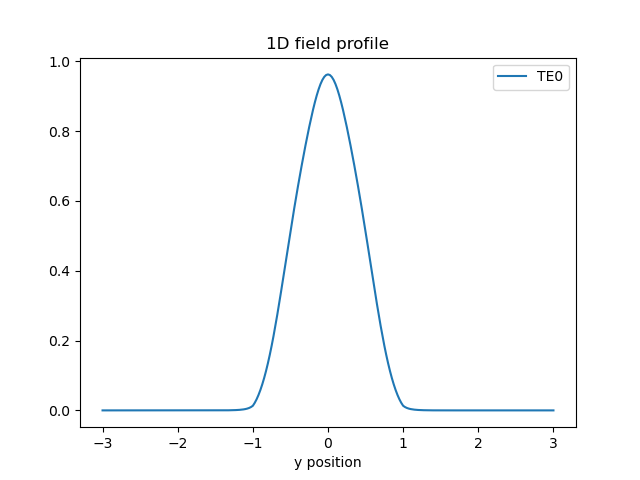
Running camfr simulation:
* Device: <LayoutCell.Layout view 'PCELL_1:layout'>
* Wavelength = 1.55 um
* Effective indices used:
- Oxide: 1.444023622
- 100nm Si: 2.1894098805166937
- 220nm Si: 2.848214685217785
Show the far field
ff = ap_sm.get_far_field(environment=environment)
ff.visualize()
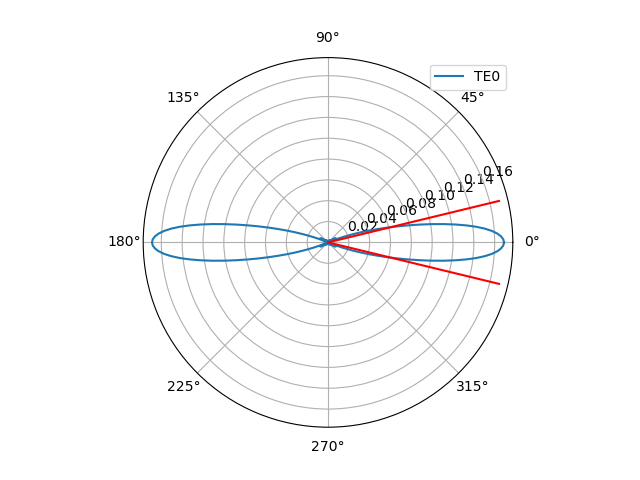
Plot the full field inside the waveguide aperture:
f2d = ap_sm.get_aperture_fields2d(environment=environment)
f2d.visualize()
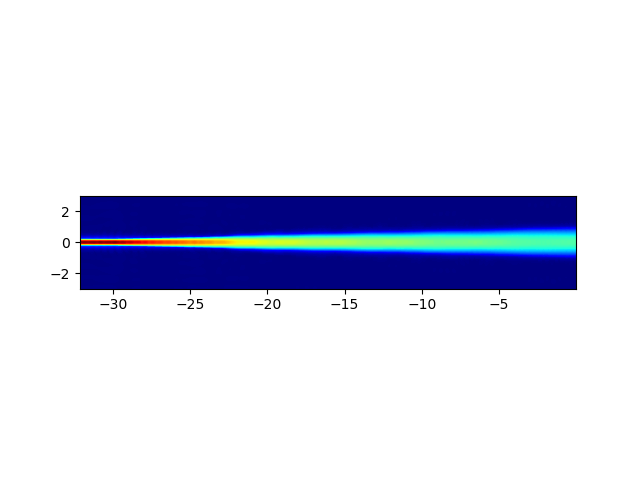
CircuitModel: Check the backreflection of the aperture
The circuitmodel will get this directly from the CAMFR simulation
ap_cm = ap.CircuitModel()
wavelengths = numpy.linspace(1.5, 1.6, 21)
s_matrix = ap_cm.get_smatrix(wavelengths=wavelengths)
Plot the reflection of the aperture.
s_matrix.visualize(
term_pairs=[("in", "in")],
scale="dB",
)
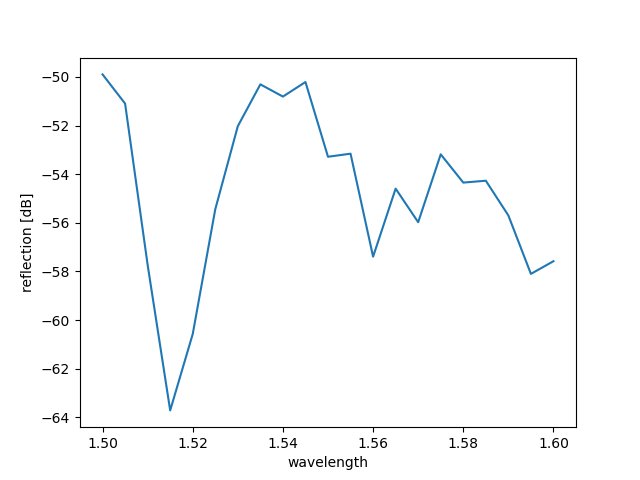
Total running time of the script: ( 4 minutes 52.373 seconds)