Join Shapes
- ipkiss3.all.join_shapes(shapes)
Joining all shapes in the shapes list after each other. Mainly used for waveguide center lines.
Angles are determined by start_face_angle or end_face_angle if defined, otherwise the last 2 points of shape(X), and the first 2 points of shape(X+1) are used.
This function only accept open shapes with more than 1 point, and will return an open shape.
- Parameters:
- shapesndarray
array of all open shapes or points needed to be joined
shapes, np.array and arrays are accepted
- Returns:
- Shape
Examples
import ipkiss3.all as i3
bend = i3.ShapeBend()
straight = i3.Shape(points=[(0,0), (2,0)])
shape = i3.join_shapes([straight, bend, straight, bend, straight])
shape.visualize()
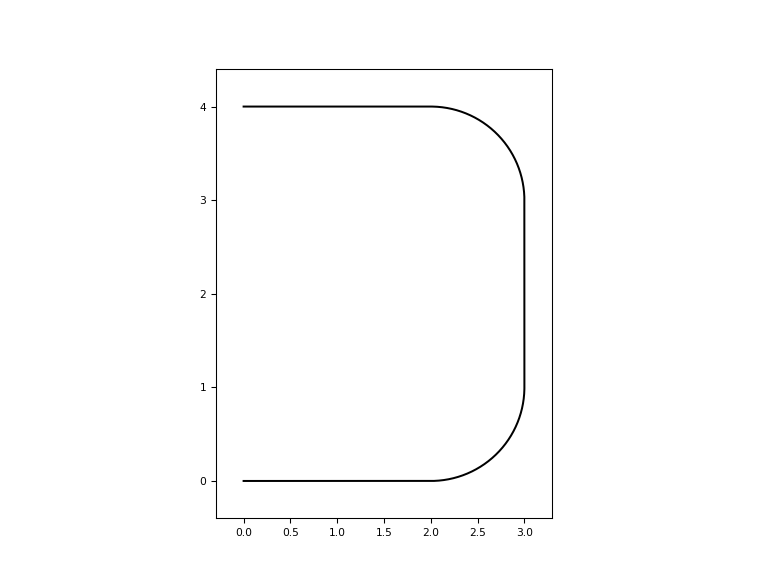
Advanced examples
When using join_shapes()
, it is important to assign the correct start_face_angle and end_face_angle, as the angle between two shapes depends on that. This can be seen in the following example:
import ipkiss3.all as i3
import matplotlib.pyplot as plt
straight = i3.Shape(points=[(0,0), (1,0)]) # flat line
angle = i3.Shape(points=[(0, 0), (1, 2)]) # line at an angle
shape = i3.join_shapes([straight, angle, straight])
shape.visualize()
plt.ylim(-1, 1)
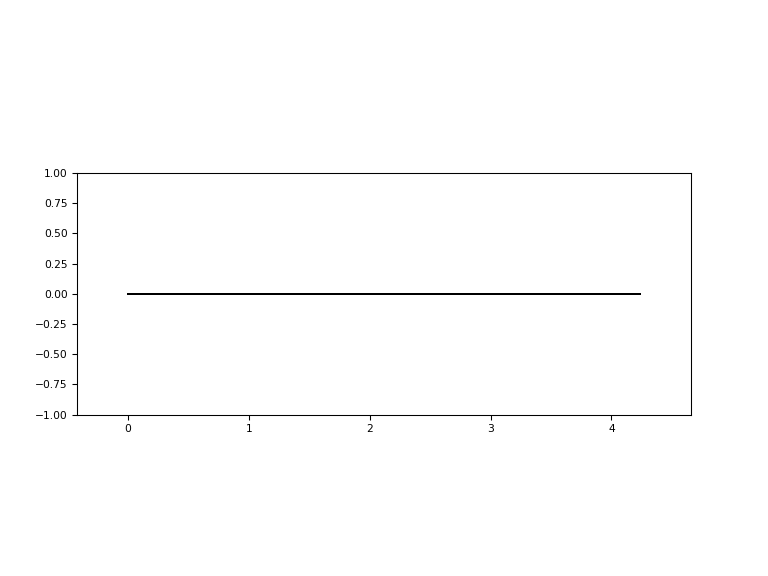
When the angle needs to be preserved, start_face_angle and end_face_angle should be defined.
import ipkiss3.all as i3
straight = i3.Shape(points=[(0,0), (1,0)])
angle = i3.Shape(points=[(0, 0), (1, 2)], start_face_angle=0, end_face_angle=0)
shape = i3.join_shapes([straight, angle, straight])
shape.visualize()
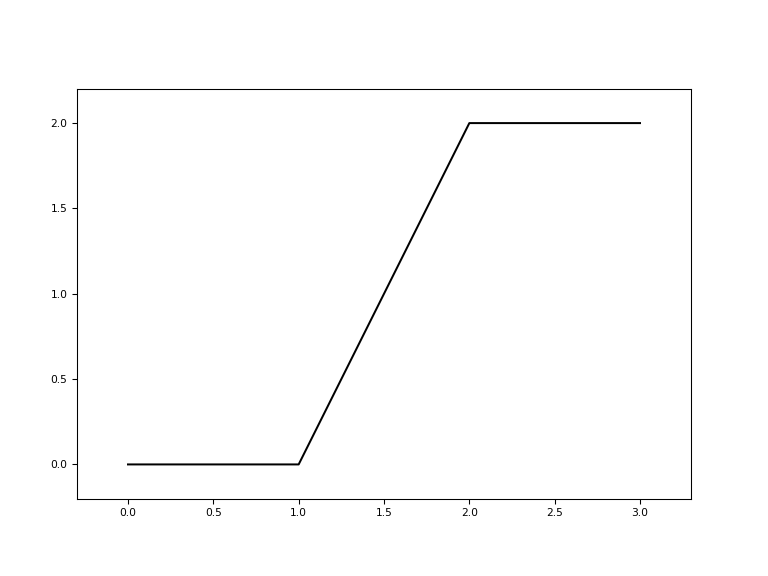