Windows
Every layer that is used inside a trace template is positioned in a certain window. You define a window with a specific start and end offset from the centerline of the trace.
Windows are usually created inside i3.WindowWaveguideTemplate
or i3.ElectricalWindowWireTemplate
.
After defining the list of windows inside your trace template, you are able to create a virtual fabrication of a cross-section with help of the technology defined inside your used PDK.
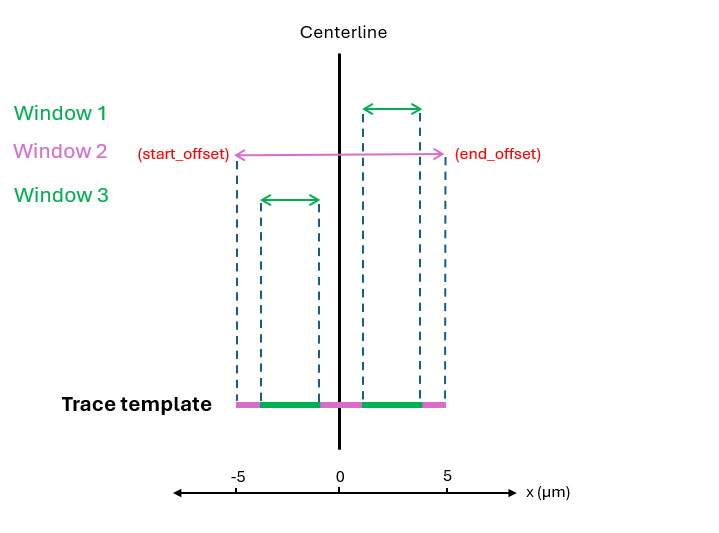
PathTraceWindow
i3.PathTraceWindow
is the most basic implementation for a window inside a trace template.
It creates a window of a specific layer at a certain start and end offset from the centerline.
- class ipkiss3.all.PathTraceWindow
Defines a window to be extruded along a shape in the form of a Path
- Parameters:
- layer: __Layer__, required
- end_offset: float, required
- start_offset: float, required
- line_width: float and int, float, integer, floating and number >= 0, optional
- shape_property_name: str and String that contains only ISO/IEC 8859-1 (extended ASCII py3) or pure ASCII (py2) characters, optional
Name of the property of the WindowTrace object that is used to generate the layout elements
Examples
import si_fab.all as pdk # noqa: F401 import ipkiss3.all as i3 class MyWgTemplate(i3.WindowWaveguideTemplate): class Layout(i3.WindowWaveguideTemplate.Layout): core_width = i3.PositiveNumberProperty(default=0.45, doc="Core width of the waveguide") cladding_width = i3.PositiveNumberProperty(default=4.0, doc="Cladding width of the waveguide") def _default_windows(self): return [ i3.PathTraceWindow( layer=i3.TECH.PPLAYER.SI, start_offset=-0.5 * self.core_width, end_offset=+0.5 * self.core_width, ), i3.PathTraceWindow( layer=i3.TECH.PPLAYER.SI_CLADDING, start_offset=-0.5 * self.cladding_width, end_offset=+0.5 * self.cladding_width, ), ] # Instantiate the new waveguide template to use it in our waveguide below wg_tmpl = MyWgTemplate() wg = i3.Waveguide(trace_template=wg_tmpl) wg_lay = wg.Layout(shape=[(0, 0), (10, 10)]) wg_lay.visualize(annotate=True)
ExtendedPathTraceWindow
i3.ExtendedPathTraceWindow
is used when you want to create a window where the layer has an extension on both sides of the trace.
- class ipkiss3.all.ExtendedPathTraceWindow
Defines a window to be extruded along a shape in the form of a path.
The shape is extended on both ends (two numbers should be given for the extension length)
- Parameters:
- layer: __Layer__, required
- end_offset: float, required
- start_offset: float, required
- extension: Coord2, optional
extension length at the beginning and end of the trace: (start length, end length)
- line_width: float and int, float, integer, floating and number >= 0, optional
- shape_property_name: str and String that contains only ISO/IEC 8859-1 (extended ASCII py3) or pure ASCII (py2) characters, optional
Name of the property of the WindowTrace object that is used to generate the layout elements
Examples
from si_fab import all as pdk from ipkiss3 import all as i3 class MyExtendedWgTemplate(i3.WindowWaveguideTemplate): class Layout(i3.WindowWaveguideTemplate.Layout): core_width = i3.PositiveNumberProperty(default=0.5, doc="width of the core section") cladding_width = i3.PositiveNumberProperty(default=4.0, doc="width of the cladding") def _default_windows(self): return [ i3.ExtendedPathTraceWindow( extension=(2.0, 4.0), layer=pdk.TECH.PPLAYER.SI, start_offset=-0.5 * self.core_width, end_offset=0.5 * self.core_width, ), i3.PathTraceWindow( layer=pdk.TECH.PPLAYER.SI_CLADDING, start_offset=-0.5 * self.cladding_width, end_offset=0.5 * self.cladding_width, ), ] trace_template = MyExtendedWgTemplate() wg = i3.RoundedWaveguide(trace_template=trace_template) wg_layout = wg.Layout(shape=[(0.0, 0.0), (10.0, 10.0)]) wg_layout.visualize()
PeriodicArrayReferenceTraceWindow
i3.PeriodicArrayReferenceTraceWindow
is used when you want to create a window for a trace template of a periodic trace.
First, you define a periodic element you want to fill your trace with and then you select the LayoutView of this element as a reference parameter of your i3.PeriodicArrayTraceWindow
.
- class ipkiss3.all.PeriodicArrayReferenceTraceWindow
Define a shape along a given path and fill it with a regular array of components
- Parameters:
- pitch: float and number > 0, required
- reference: _LayoutView, required
- exclude_ends: Coord2 and number >= 0, optional
- offset: float, optional
- shape_property_name: str and String that contains only ISO/IEC 8859-1 (extended ASCII py3) or pure ASCII (py2) characters, optional
Name of the property of the WindowTrace object that is used to generate the layout elements
Examples
from ipkiss3 import all as i3 from si_fab import all as pdk # Create our periodic element, the SubwavelengthCell class SubwavelengthCell(i3.PCell): class Layout(i3.LayoutView): core_layer = i3.LayerProperty(default=i3.TECH.PPLAYER.SI) cell_length = i3.PositiveNumberProperty(default=4, doc="length of the structures [um]") cell_width = i3.PositiveNumberProperty( default=2, doc="width of the structures [um] (default is period * (1 - filling_factor))", ) def _generate_elements(self, elems): elems += i3.Rectangle( layer=self.core_layer, center=(0.0, 0.0), box_size=(self.cell_width, self.cell_length), ) return elems # Waveguide template class SubwavelengthGratingTemplate(i3.WindowWaveguideTemplate): class Layout(i3.WindowWaveguideTemplate.Layout): cladding_width = i3.PositiveNumberProperty(default=4, doc="cladding width (um)") core_width = i3.PositiveNumberProperty(default=4, doc="core width [um]") period = i3.PositiveNumberProperty(default=1, doc="period of the structures [um]") fill_factor = i3.FractionProperty(default=0.5, doc="filling factor of structures [0, 1]") cell_length = i3.PositiveNumberProperty(default=4, doc="length of the structures [um]") cell_width = i3.PositiveNumberProperty(doc="width of the structures [um]") def _default_cell_width(self): return round((1 - self.fill_factor) * self.period, 3) def _default_core_layer(self): return pdk.TECH.PPLAYER.SI def _default_windows(self): exclusion = self.cell_width / 2.0 subwavelength_cell = SubwavelengthCell() subwavelength_cell.Layout( core_layer=self.core_layer, cell_length=self.cell_length, cell_width=self.cell_width, ) return [ i3.PeriodicArrayReferenceTraceWindow( reference=subwavelength_cell, offset=0, exclude_ends=(exclusion, exclusion), pitch=self.period, ), i3.PathTraceWindow( layer=pdk.TECH.PPLAYER.SI_CLADDING, start_offset=-0.5 * self.cladding_width, end_offset=0.5 * self.cladding_width, ), ] # Create subwavelength grating trace_template = SubwavelengthGratingTemplate() wg = i3.RoundedWaveguide(trace_template=trace_template) wg_layout = wg.Layout(shape=[(0.0, 0.0), (20.0, 0.0), (20.0, 20.0)]) wg_layout.visualize()